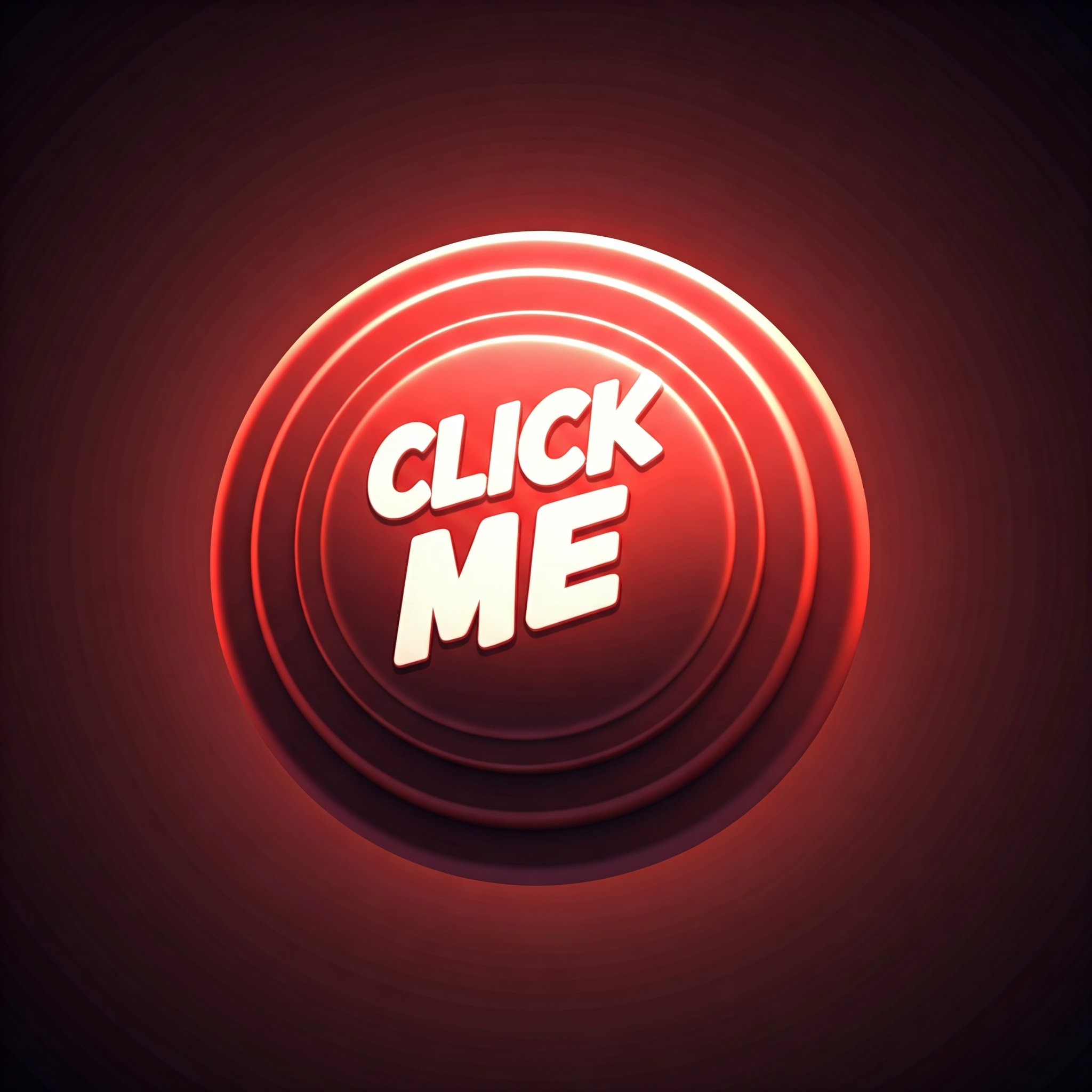
This blog post will guide you through the process of designing and developing an interactive button, starting with a visual representation in Sketch and translating it into functional HTML and CSS using Tailwind CSS.
1. Designing in Sketch
- Create a New Artboard: Start by creating a new Artboard in Sketch. This will serve as your canvas for the button design.
- Shape and Size: Use the Rectangle tool to create the button's shape. Adjust the width, height, and corner radius to achieve the desired appearance.
- Fill and Stroke: Apply the desired background color and border (if any).
- Text: Add the button text using the Text tool. Choose a suitable font, size, and color.
- Hover State: Create a duplicate of the button layer. This will represent the button's appearance when hovered over. Modify the hover state with subtle changes like a slightly darker background color, a subtle shadow, or a slight increase in size.
2. Coding in Tailwind CSS
Now, let's translate our Sketch design into functional HTML and CSS using Tailwind CSS.
Basic Structure:
HTML
<button
class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded"> Click Me
</button>
Breakdown:
bg-blue-500
: Sets the background color of the button to a shade of blue.hover:bg-blue-700
: Changes the background color to a darker shade of blue on hover.text-white
: Sets the text color to white.font-bold
: Makes the text bold.py-2 px-4
: Sets padding for the button (vertical and horizontal).rounded
: Applies rounded corners to the button.
3. Adding Interactivity (Optional)
- JavaScript (for more complex interactions): You can use JavaScript to add more advanced interactions, such as:
- Click events: Trigger actions like form submissions or displaying modals.
- Animations: Create more sophisticated hover effects or transitions using JavaScript libraries like GSAP.
Example with JavaScript:
HTML
<button class="bg-blue-500 hover:bg-blue-700 text-white font-bold py-2 px-4 rounded" onclick="showAlert()">Click Me</button>
<script>
function showAlert() {
alert("Button clicked!");
}
</script>
Key Considerations:
- Accessibility: Ensure your button is accessible to all users, including those with disabilities. Use appropriate ARIA attributes (e.g.,
role="button"
) and consider keyboard navigation. - Responsiveness: Design your button to look good and function properly on different screen sizes and devices.
- Maintainability: Use a consistent naming convention for your classes to improve code readability and maintainability.
Conclusion
By following these steps, you can effectively translate your Sketch designs into interactive and visually appealing buttons using Tailwind CSS. Remember to prioritize user experience and accessibility while exploring the creative possibilities of both Sketch and Tailwind CSS.
This guide provides a basic framework. Feel free to experiment with different colors, styles, and interactions to create unique and engaging button designs.